今回は、OpenCVでフィルタを使って画像のエッジ抽出をしてみました。
エッジ抽出に使われるフィルタはPrewittフィルタやSobelフィルタ、ラプラシアンフィルタなどいくつか種類がありますが、今回はSobelフィルタを使ってみます。
目次
今回の環境
・OS : Windows10(64bit)
・Visual Studio 2015 Community
・OpenCV 4.2.0
(上記全て環境構築済)
今回はOpenCVは環境済の状態でスタートするので、OpenCVの環境構築は以下の記事などを参照してください。
過去記事①:Nugetを使ってOpenCVの環境構築(Visual Studio 2015)
過去記事②:Visual Studio 2015でOpenCV 3.4環境構築(Windows10)
Sobelフィルタとは
Sobelフィルタは以下の図のようなフィルタで、特定方向の輪郭抽出が可能なフィルタです。
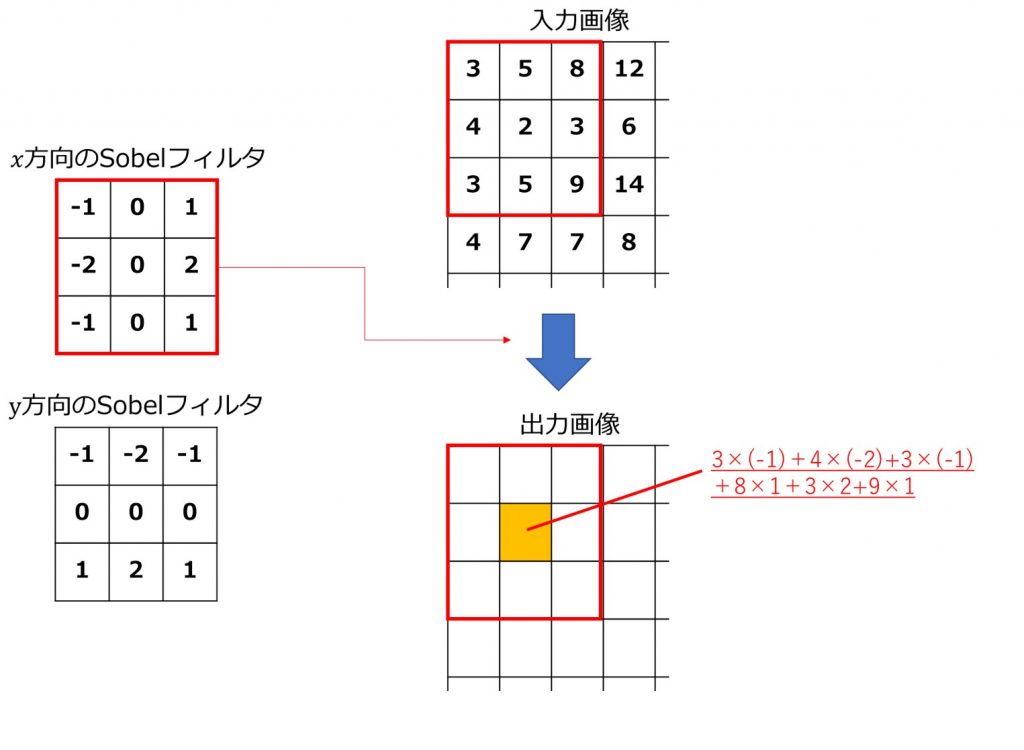
-1、0、1のように特定の方向に正負の重みづけをすることで、出力画像では画素値が大きく変化している部分の絶対値が大きくなるため、エッジを抽出することが可能です。
類似するフィルタとして、Prewittフィルタ(Sobelフィルタと同じ1次微分フィルタ)、ラプラシアンフィルタ(2次微分フィルタ)があります。
今回はこのSobelフィルタをOpenCVとC++で実装してみました。
ソースコード
以下に、Sobelフィルタでエッジを求めるプログラムを示します。
OpenCVには以下のようなSobel関数が実装されているため、非常に簡易にSobelフィルタを用いたエッジ抽出が可能になります。
また、Sobelフィルタを掛けると画素値は負になる可能性もあるため、以下のように最終的には絶対値を取って正規化することで、8bitの画素値に変換します。
以下が上記のソースコードでx方向のSobelフィルタを掛けてみた結果です。
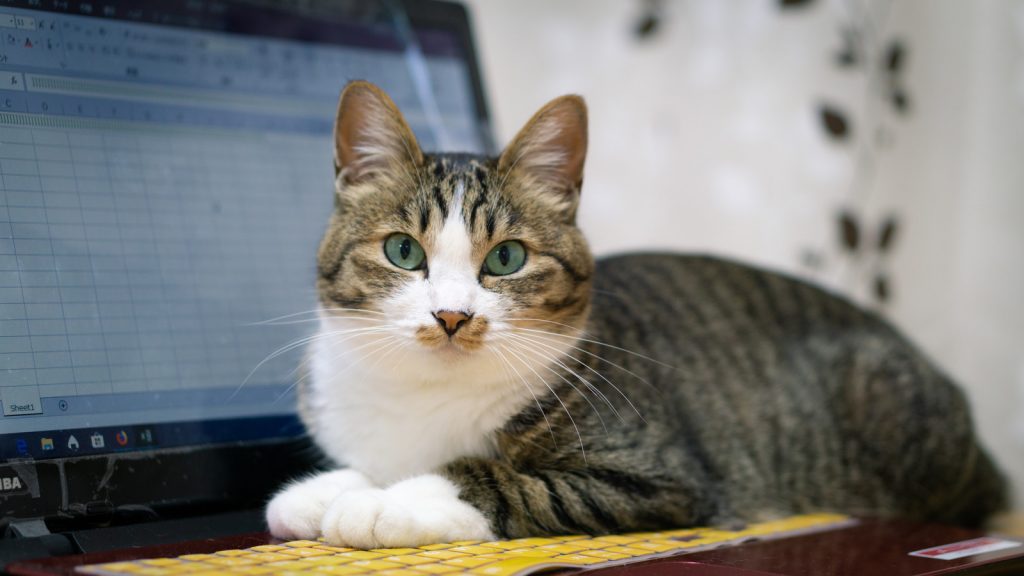
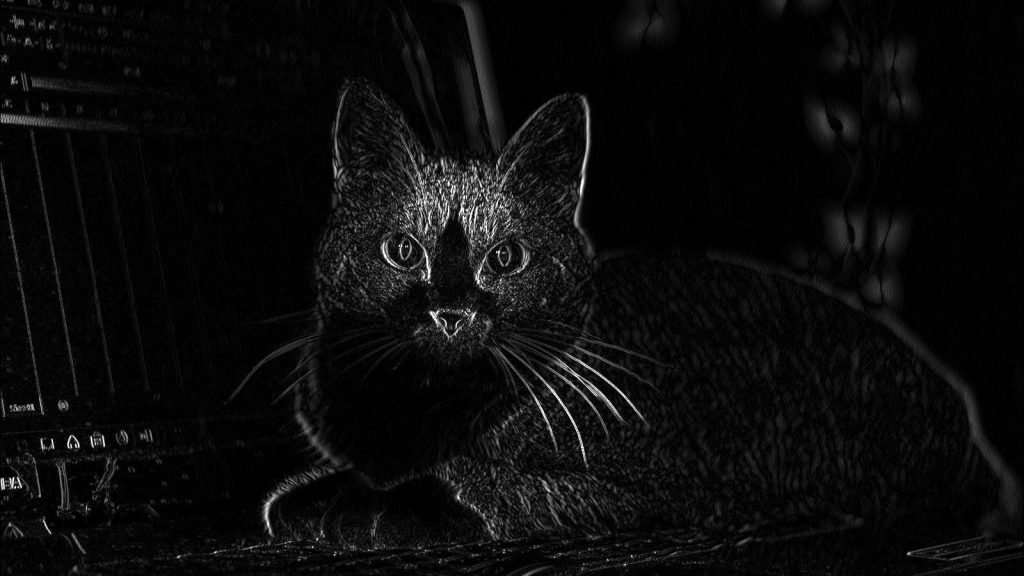
確かにエッジが抽出できていることがわかります。
まとめ
今回はOpenCVでエッジの抽出を実施してみました。
他にエッジ抽出ができるフィルタとしてラプラシアンフィルタを紹介した記事もありますので、興味のある方はこちらもご覧ください。