3Dデータ処理ができる手軽なライブラリとして、Open3Dがあります。
本日はこのOpen3Dを用いて、3Dメッシュ(ポリゴン)を読み込み、その枚数を減らすプログラムを紹介します。
Open3Dを用いることで、容易にポリゴンの枚数を減らすことができます。
もちろん形状は粗くなってしまいますが、枚数を減らすことでデータサイズの削減や、その後の処理を軽量に実施することができます。
Open3Dの環境構築に関しては、以下の環境構築に関する記事をご覧ください。
PLYファイルの入手
PLYファイルは3D点群データや3Dメッシュを保存するための汎用フォーマットで、広く用いられています。
PLYファイルで保存された3Dメッシュはさまざまなサイトで公開されていますが、今回は以下のサイトで公開されているスタンフォードバニーのPLYファイルを読み込み、実験を行いました。
外部サイト:3D Models
ポリゴンリダクションプログラム(Open3DとPythonによる実装)
実行環境
- OS:Windows 10
- Python 3.9
- Open3D ver. 0.15.1
ソースコード
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import open3d as o3d
import numpy as np
if __name__ == "__main__":
# Loading mesh data
print("Loading mesh data")
Mesh = o3d.io.read_triangle_mesh("bunny.ply")
# Calculation of normal vector
Mesh.compute_vertex_normals()
print(np.asarray(Mesh.triangle_normals))
# Mesh decimation
MeshSimple = Mesh.simplify_quadric_decimation(target_number_of_triangles=10000)
# Confirmation
print(Mesh)
print(MeshSimple)
# Visualization in window
o3d.visualization.draw_geometries([Mesh],mesh_show_back_face=True)
o3d.visualization.draw_geometries([MeshSimple],mesh_show_back_face=True)
# Saving mesh
o3d.io.write_triangle_mesh("MeshSimple.ply", MeshSimple)
コードの解説
メッシュデータの読込
メッシュの読み込みに必要なのは以下の箇所で、「io.read_triangle_mesh」を用いて読み込みたいメッシュデータのパスを指定することで読み込みが可能です。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Loading mesh data
print("Loading mesh data")
Mesh = o3d.io.read_triangle_mesh("bunny.ply")
メッシュの法線の計算
メッシュの表示を行う際に、法線ベクトルがないと陰影が付かず、表示結果が綺麗になりません。そこで、以下の箇所でメッシュの法線を計算しています。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Calculation of normal vector
Mesh.compute_vertex_normals()
print(np.asarray(Mesh.triangle_normals))
ポリゴンリダクション
以下の箇所でポリゴンの数を減らします。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Mesh decimation
MeshSimple = Mesh.simplify_quadric_decimation(target_number_of_triangles=10000)
Open3Dには、ポリゴンリダクションを実現できる関数として「simplify_quadric_decimation関数」が標準で実装されています。
引数として、リダクション後のポリゴンの枚数を指定することができます。上記のプログラムでは「10000」を設定しているため、リダクション後のポリゴンの枚数が10000枚になります。
メッシュの表示
最後に以下の箇所でメッシュデータを表示します。今回はリダクションを行わないもの、行ったものの計2回ウィンドウを表示するようにしています。
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Visualization in window
o3d.visualization.draw_geometries([Mesh],mesh_show_back_face=True)
o3d.visualization.draw_geometries([MeshSimple],mesh_show_back_face=True)
実行結果
「target_number_of_triangles」を変更して、いくつかリダクション結果を出力してみました。さすがに100ポリゴンまで減らすと原型を留めていないですが、まずまず上手く枚数を減らせているように見えます。
リダクション無し(69451ポリゴン)
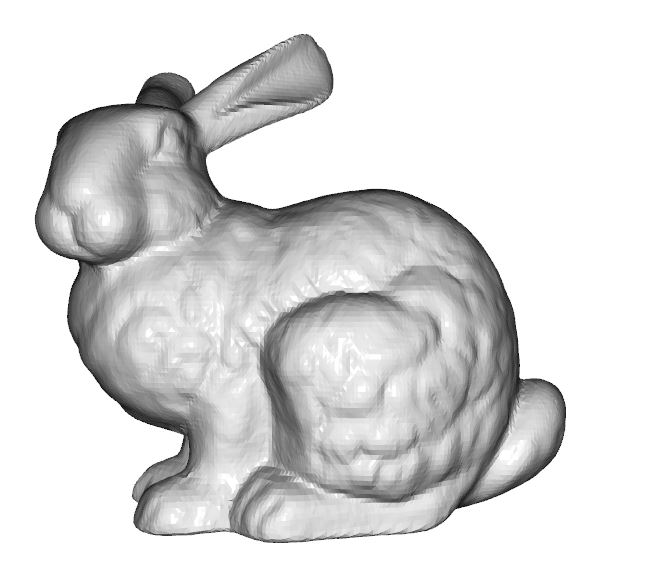
ポリゴンリダクション結果(target_number_of_triangles=10000ポリゴン)
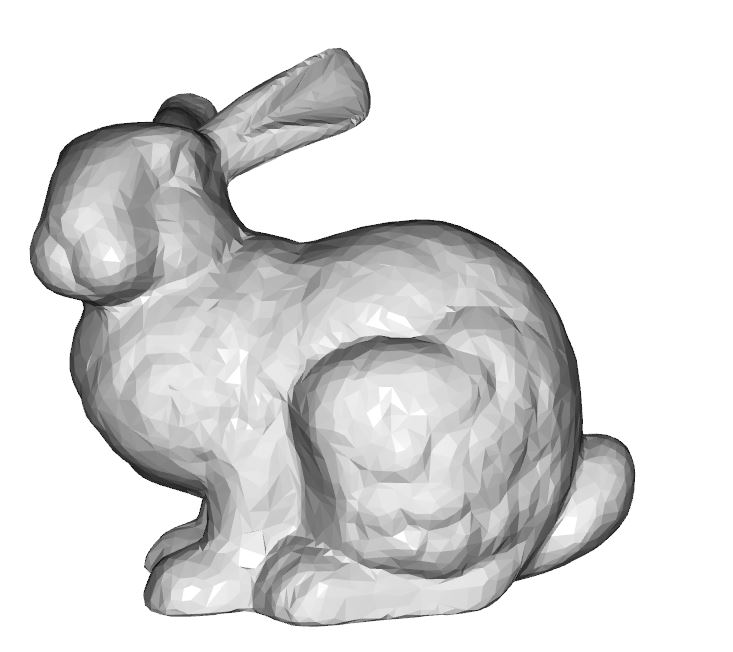
ポリゴンリダクション結果(target_number_of_triangles=1000ポリゴン)
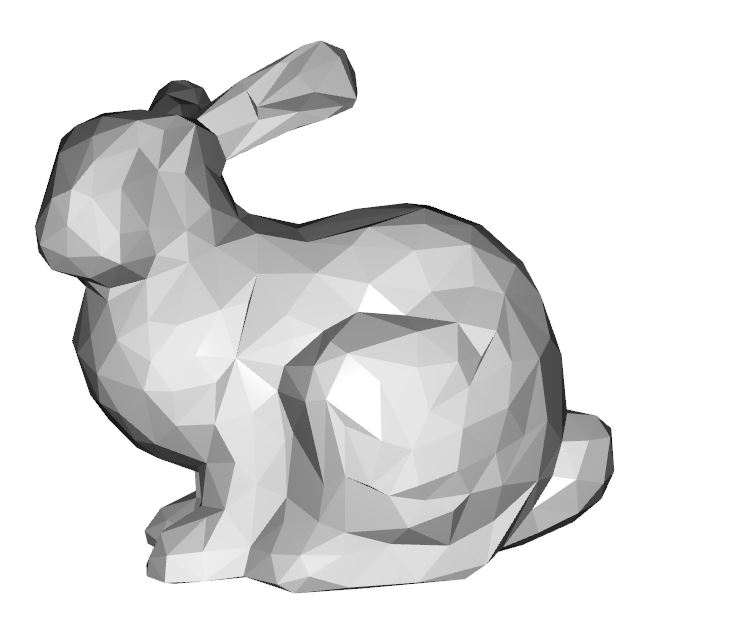
ポリゴンリダクション結果(target_number_of_triangles=100ポリゴン)
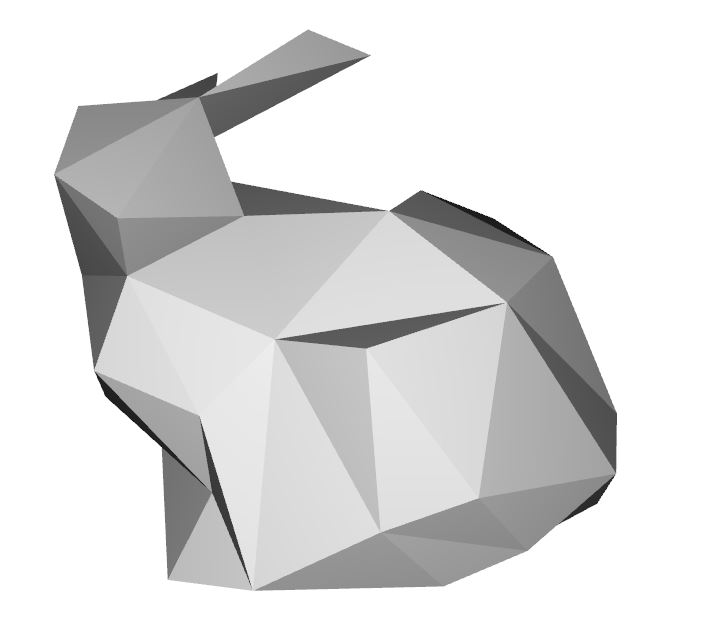
まとめ
Open3Dでポリゴンの枚数を減らす方法を紹介しました。
アプリケーションの要求に対して、ポリゴンの枚数が多すぎる場合などには、リダクションでデータを減らすことが有効です。