これまで画像のノイズ除去ができるフィルタとして、
の4つのフィルタを紹介しました。
それぞれの詳細は、上記のリンクから各フィルタの記事に飛べるので、そちらを参照してください。
この記事では、上記の4種類のフィルタの性能比較をし、どのフィルタを使うのがベターなのかを検証してみたいと思います。
目次
画像のノイズ除去ツール(Python)
4種類のフィルタの検証ができるプログラムは以下に紹介します。
動作環境:OpenCV 4.5.5
平均値フィルタはコードの以下の箇所が該当します。
ガウシアンフィルタはコードの以下の箇所が該当します。
メディアンフィルタはコードの以下の箇所が該当します。
バイラテラルフィルタはコードの以下の箇所が該当します。
比較結果
今回テストデータとして以下の画像と用いました。
上が正解画像、下がノイズ画像となります。下のノイズ画像に対して処理を施し、正解画像にどれだけ近づけたかを評価します。
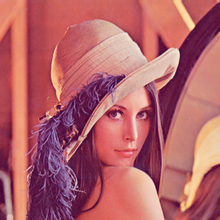
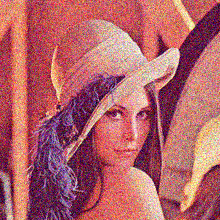
評価方法としてはPSNRとSSIMを採用しました。
定量評価結果
各フィルタに関して、少しずつウィンドウサイズを広げて比較を行いました。
バイラテラルフィルタのみ9×9まで広げても精度劣化が見られなかったため、最終的に11×11まで評価しました。それ以降13×13より大きくしても精度が改善しないことを確認しています。
バイラテラルフィルタのパラメータはsigmaColor=100, sigmaSpace=10としました。
結果を以下の表に示します。
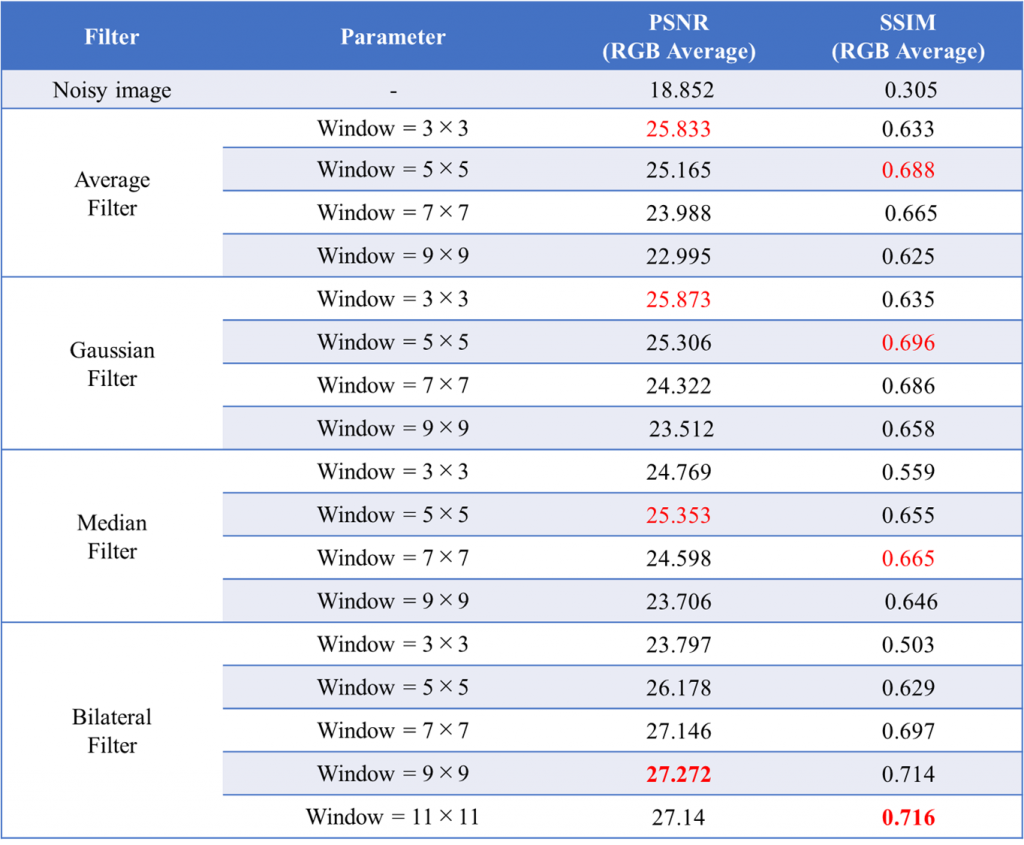
赤字は各フィルタの中での最高値を示します。ウィンドウサイズが大きすぎると精度が低下する傾向にあり、平均値フィルタ、ガウシアンフィルタ、メディアンフィルタは3×3~7×7あたりで最高値を示しました。
一方バイラテラルフィルタはフィルタのサイズを大きめに取った方が良く、9×9や11×11が良い結果を示しました。
また、各フィルタ間の比較においてはバイラテラルフィルタが一番良い結果を示しました。
見た目についても確認してみましょう。PSNRやSSIMの結果にも表れているように、9×9のバイラテラルフィルタが一番良い結果のように見えます。
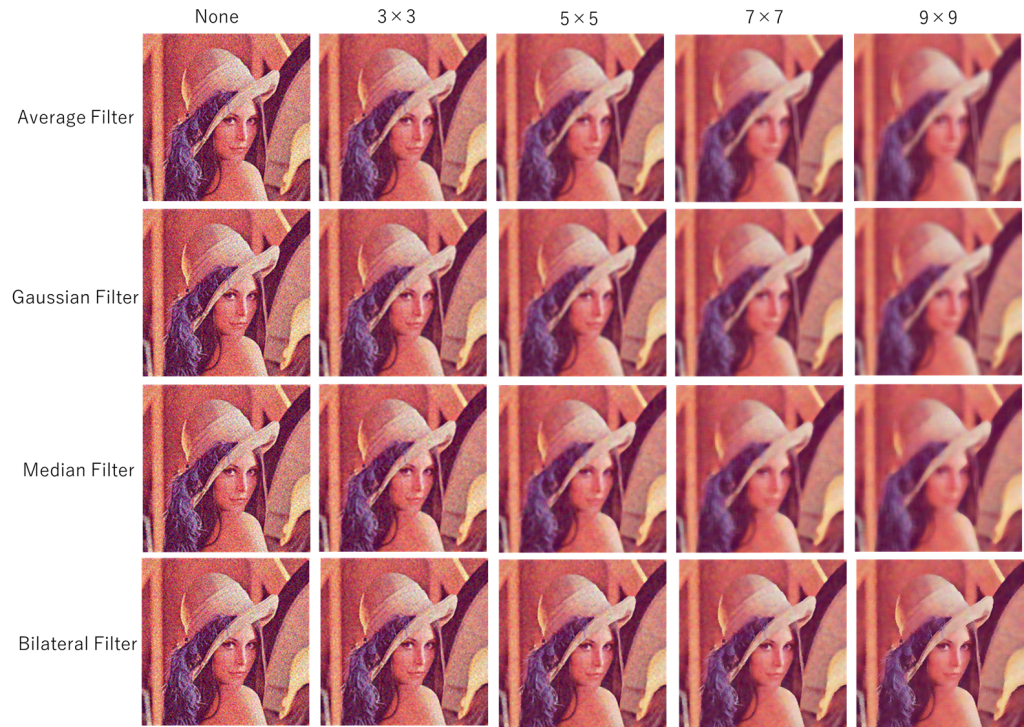
まとめ
4種類のフィルタの比較を行いました。
結論としては、バイラテラルフィルタを使うのが、一番安定して良い結果を得ることができそうです。処理時間の問題等がなく、OpenCVに標準実装されているフィルタで簡易にノイズ除去をしたい場合には、まずはバイラテラルフィルタを試してみるのが良さそうですね。