今回は、OpenCV等に実装されている3種類のエッジ抽出フィルタ
- Prewittフィルタ
- Sobelフィルタ
- Laplacianフィルタ
の3種類のフィルタを試し、その効果を比較・検証してみたいと思います。
目次
各フィルタの詳細
各フィルタの詳細については、過去の記事にて紹介しておりますので、それぞれのページをご覧ください。いずれもエッジ抽出効果のあるフィルタとなります。
エッジ抽出効果検証用プログラム(Pythonでの実装)
画像に各フィルタを適用するプログラムを以下で紹介します。
ソースコード
動作環境:OpenCV 4.5.5
エッジ抽出結果
あまり大きな差はないですが、Sobelフィルタが一番エッジがくっきり太く現れている感じがします。一方、ラプラシアンフィルタで抽出されるエッジは細線化される傾向にありました。
Prewittフィルタ
上が入力画像、下がフィルタ適用結果です。
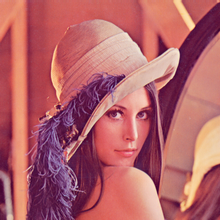
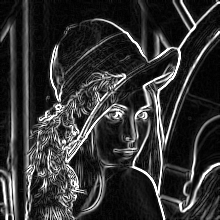
Sobelフィルタ
上が入力画像、下がフィルタ適用結果です。
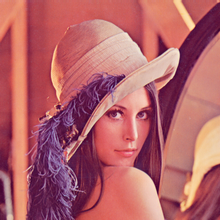
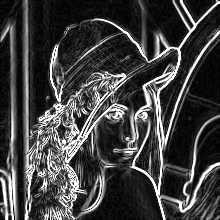
Laplacianフィルタ
上が入力画像、下がフィルタ適用結果です。
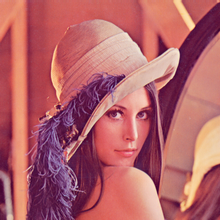
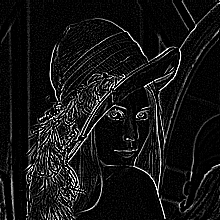
まとめ
3種類のエッジ強調フィルタについて、結果の比較を行いました。どのフィルタを用いてもエッジ抽出自体はできそうですが、出力結果に関しては違いがあるため、アプリケーションに応じて適したフィルタを選択する必要がありそうです。